“Only integer scalar arrays can be converted to a scalar index“, you will get this error message when you try to combine multiple arrays into a single array in python using NumPy.
NumPy is a fundamental python library that is for scientific computing in python. Numpy provides a multidimensional array object which gives great control to perform mathematical operations over the data. This error message comes when you tried to handle data using array objects. Don’t worry, it’s a very simple and basic error message.
Go through this article thoroughly to understand why you are getting this error message and how to resolve it. So don’t waste your time and start understanding the problem.
Why you are getting only integer scalar arrays can be converted to a scalar index error message?
If you see the error message then it is a little bit confusing and does not give detailed information about the actual error. So don’t break your head here trying to understand the error message. In the below example, we have reproduced the error message. Based on this example we will try to understand and resolve the error message.
# Sample Program.....................
# Created By: Technolads.com.........
# File Name: Sample_Program_1.py
#import numpy
import numpy
# Create 3 array and store required values
array_1 = numpy.array(['t', 'e', 'c'])
array_2 = numpy.array(['h', 'n','o'])
array_3 = numpy.array(['l', 'a','d','s'])
# Concatenate array_1, array_2 and array_3 into array_4
array_4 = numpy.concatenate(array_1, array_2, array_3)
print(array_4)
When you try to execute the above program using a command prompt, you will get the below error message.
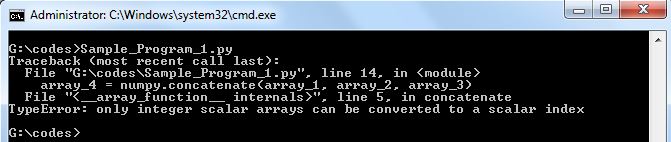
in the error message, it is mentioned that there is an error at line number 14 in the code i.e. following line of the code
array_4 = numpy.concatenate(array_1, array_2, array_3)
It means it’s giving an error while trying to concatenate the multiple arrays into a single array. But why? We are just simply concatenating the arrays. Let’s understand this.
Numpy’s concatenation function concatenates tuple or list data types but not an array. And in this example, we are trying to concatenate multiple arrays that’s why we are getting this error message.
How to fix only integer scalar arrays can be converted to a scalar index error?
Now we understood that Numpy’s concatenates function concatenates tuple and list data type only. So here we have to convert an array into a list or tuple. Let’s first understand what is tuple and list.
Tuple: Tuples are immutable data structures that contain elements of different data types. You cannot modify the elements of the tuple. Tuple does not come with many inbuilt functions, So it is not handy to process data stored in the tuple
List: Lists are mutable data structures that can store elements of different data types. You can modify the elements of the List. The list comes with many inbuilt functions which can be used to process data easily.
You can convert an array to a tuple by adding comma-separated array values in square brackets shown below:
array_4 = numpy.concatenate([array_1, array_2, array_3])
You can convert array to a list by adding comma-separated array values in round brackets shown below:
array_4 = numpy.concatenate((array_1, array_2, array_3))
If you make any one of the above changes in our example and re-run the code then you will get the following output on the command prompt.

Final Thoughts:
In this article, we tried to understand the reason behind the “Only integer scalar arrays can be converted to a scalar index” error message and its solution. We hope you are able to get rid of this error message by changing an array data type to list or tuple. Please share your experience or views in the comment section or you can reach out to us using the contact form.