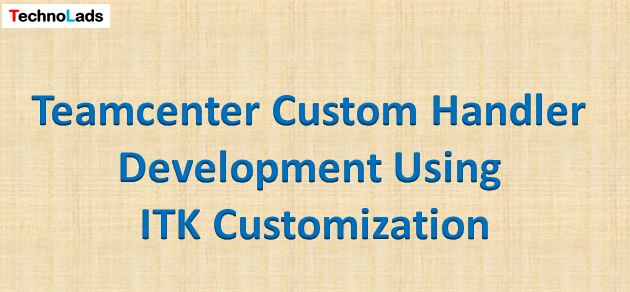
Teamcenter workflow designer is one of the most important modules of Teamcenter software.
The workflow process is a sequence of operations performed for validation, change, and approval. We can develop a workflow template using the workflow designer application of the Teamcenter software. You must be an administrative user to create a custom workflow template.
Workflow handlers are very important while designing the workflow template. Handlers are used to perform required action or required validation on the workflow attachments at different stages of the workflow process. Siemens has provided some default handlers that we can use while designing the workflow template. But sometimes these OOTB handlers alone can’t satisfy the specific business process requirement.
Siemens has given the flexibility to develop custom handlers using ITK customization to meet specific business process requirements in their PLM application. In this article, we are going to study the development of custom handlers using ITK customization.
What Are The Different Types of Handlers Available in the Teamcenter?
There are mainly two types of handlers available in the workflow designer application of the Teamcenter. i.e. Action Handler and Rule Handler.
Action Handler
Action handler performs required actions such as set property value on the target object, create form, attach objects to the target object with required relation, etc.
Examples: EPM-create-form, EPM-demote, EPM-attach-related-objects, EPM-auto-assign
Rule handler
Rule handler validates that the required condition is satisfied before completing the task or performing any action on the target objects. Such as the user should fill in required attribute information on the target objects before completing the task or the user should not remove any objects from target attachments.
Examples: EPM-check-item-status, EPM-check-object-properties, EPM-check-related-objects
Basic Template For Action Handler Development
Following is the basic code template that you can use for the development of a custom action handler. EPM_register_action_handler ITK API registers your custom handler.
extern DLLAPI int customDLL_register_callbacks()
{
int ifail = ITK_ok;
ifail = CUSTOM_register_exit("customDLL","USER_gs_shell_init_module",
(CUSTOM_EXIT_ftn_t),register_custom_code);
return ifail;
}
extern DLLAPI int register_custom_code(int* decision, va_list args)
{
int ifail = ITK_ok;
*decision = ALL_CUSTOMIZATIONS;
ifail = EPM_register_action_handler("technolads","technical
website",technolads_custom_function);
return ifail;
}
int technolads_custom_function(EPM_action_message_t message)
{
int ifail = ITK_ok;
// Your required custom logic
return ifail;
}
Basic Template For Rule Handler Development
Following is the basic ITK code template that you can use for the development custom rule handler. EPM_register_rule_handler ITK API registers your custom handler.
The return type of rule handler is EPM_decision_t. If a condition is satisfied then the rule handler returns EPM_go command, allowing the task to continue. If a condition is not satisfied then it returns EPM_nogo command.
extern DLLAPI int customDLL_register_callbacks()
{
int ifail = ITK_ok;
ifail = CUSTOM_register_exit("customDLL","USER_gs_shell_init_module",
(CUSTOM_EXIT_ftn_t),register_custom_code);
return ifail;
}
extern DLLAPI int register_custom_code(int* decision, va_list args)
{
int ifail = ITK_ok;
*decision = ALL_CUSTOMIZATIONS;
ifail = EPM_register_rule_handler("technolads","technical website",
(EPM_rule_handler_t)technolads_custom_function);
return ifail;
}
EPM_decision_t technolads_custom_function(EPM_rule_message_t message)
{
EPM_decision_t decision = EPM_go;
// Your required logic
return decision;
}
How to Deploy Custom Handler In The Teamcenter Environment?
When you compile and build your custom handler code then one dll gets generated. You need to place this dll into the TC_ROOT\bin folder.
Add the name of your custom dll into the TC_customization_libraries preference.
Restart the Teamcenter application. You will see a custom handler in the handler attachment section while editing the workflow template in the workflow designer.
Please check 10 examples of Teamcenter Custom Handlers development using ITK customization article. You will understand actual industry real-life scenarios of custom handler development.
You can also refer to our following articles regarding ITK customization.
What Did You Learn In This Article?
To summarise, in this article we have learned the following points:
- Basics of action handler and rule handler
- Need of Teamcenter custom handler development
- The basic code template for the development of a custom action handler
- The basic code template for the development of a custom rule handler
- Steps to deploy custom handler in Teamcenter environment
We hope this article was informative and you got a clear idea about Teamcenter custom handler development using ITK customization. If you face any challenge or need more information then please do mention it in the comment section or you can reach out to us using the contact form.